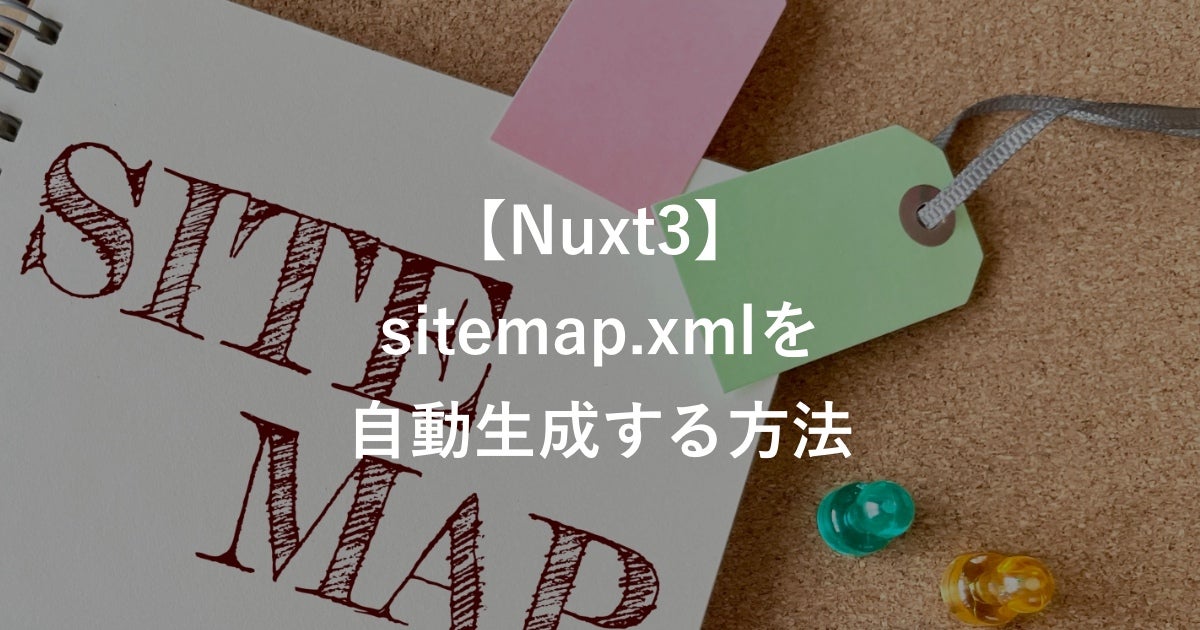
How to Automatically Generate sitemap.xml in Nuxt 3
- Technology
- Independent Development
- Nuxt.js
Overview
In this article, I will summarize the method for implementing the automatic generation of sitemap.xml in my portfolio site built with Nuxt 3. While Nuxt provides the ability to generate sitemap.xml using packages like "@nuxtjs/sitemap," there wasn't a solution available for Nuxt 3 or SSR (Server-Side Rendering). Therefore, I decided to create this functionality myself. The Nuxt version used in this implementation is "3.0.0-rc.10."
Implementation
In Nuxt, you can easily create APIs by creating files under the "/server" directory. To utilize this feature, I first created a file named "sitemap.xml.ts" under "/server/routes." Typically, files under the "/server/api" directory automatically get routed with the "/api" prefix. However, if you want to add server routes with a different prefix, you can place them in the "/server/routes" directory. This way, when you access "/sitemap.xml," it will return the result of the processing in "server/routes/sitemap.xml.ts."
All that's left is to write the code in "sitemap.xml.ts" to output the contents of the sitemap.
export default defineEventHandler(async (event) => {
const sitemapInfos: {
loc: string;
lastmod: string;
priority: string;
}[] = [
{
loc: 'https://sample.com/',
lastmod: '2022-10-05T18:35:28+09:00',
priority: '1.00',
},
];
let sitemapString = `<?xml version="1.0" encoding="UTF-8"?>
<urlset
xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.sitemaps.org/schemas/sitemap/0.9
http://www.sitemaps.org/schemas/sitemap/0.9/sitemap.xsd">
`;
for (let i = 0, iLength = sitemapInfos.length; i < iLength; i = (i + 1) | 0) {
const sitemapInfo = sitemapInfos[i];
sitemapString += `
<url>
<loc>${sitemapInfo.loc}</loc>
<lastmod>${sitemapInfo.lastmod}</lastmod>
<priority>${sitemapInfo.priority}</priority>
</url>
`;
}
sitemapString += `</urlset>`;
event.res.setHeader('content-type', 'text/xml');
event.res.end(sitemapString);
});
In this code, we essentially create the contents of the XML as a string and then return it. The key points are setting the response headers with "event.res.setHeader" and sending the XML using "event.res.end." For more details, you can refer to the source code on GitHub.