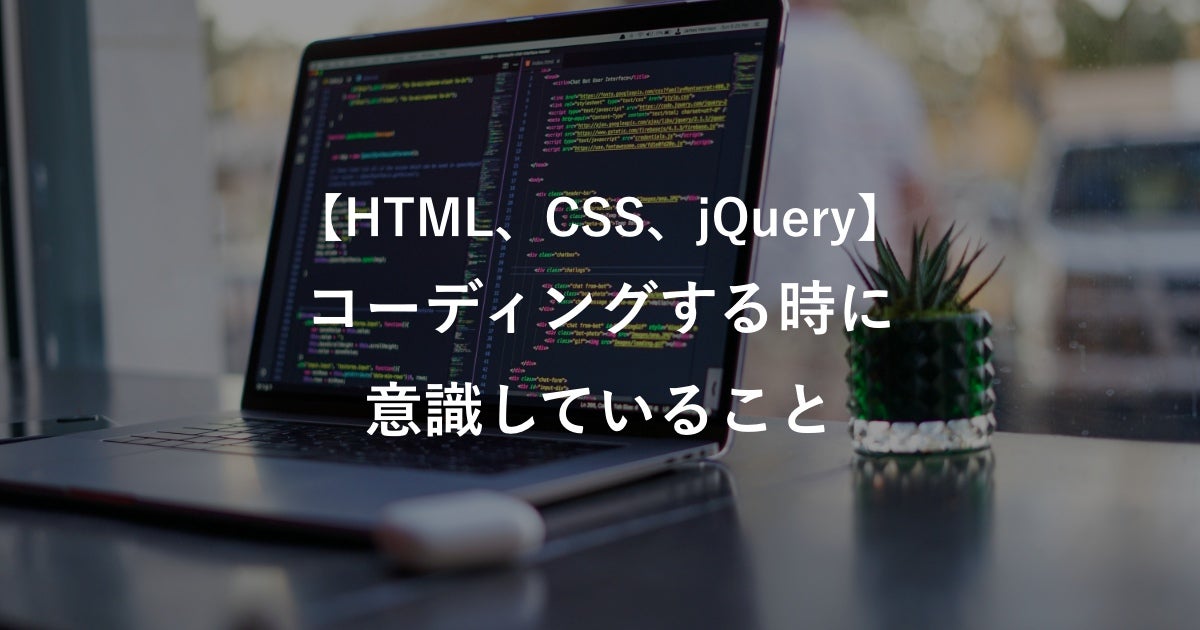
[HTML, CSS, jQuery] Things to Keep in Mind When Coding
Post Date
- Tips
- HTML
- CSS
- JavaScript
- jQuery
Overview
In this article, I will summarize the principles I adhere to while coding, with a focus on using HTML, CSS, and JavaScript (jQuery) as the assumed programming languages.
HTML
Be conscious of the heading levels for each page
HTML provides h1 to h6 header tags.
Strive to set them appropriately.
- Use h1 for the main visual catchphrase or header logo on the homepage.
- For subpages, designate the page title as h1.
- If there are titles within page sections, use h2.
- Use h3 and beyond when necessary, considering design elements.
Tag elements thoughtfully based on their meaning
- Utilize ul for regular lists and ol for ordered lists.
- Create clickable elements using buttons.
- …etc
Maintain consistent and meaningful class names
- Follow naming conventions such as BEM or kebab-case.
- Avoid mixing abbreviations and non-abbreviated forms of the same word (e.g., 'text' and 'tx').
CSS
Create styles with broad applicability
- Prefer single selectors that are not overly dependent on parent or descendant elements.
.Hoge {
~
}
.Fuga {
~
}
- Use class names over HTML tags in selectors
.HogeLink {
~
}
Efficiently manage colors
- Use Sass or CSS variables to easily track the colors used within the site.
Standardize units for values
- Define unit rules (e.g., rem for font-size and px for margins) and adhere to them throughout coding.
jQuery
Develop versatile functionalities
- When manipulating the DOM, avoid using attributes from CSS or other styles for selectors. Assign dedicated classes like 'JsSample' for reference.
Prioritize lightweight solutions
- For repetitive tasks, prefer native JavaScript constructs like 'for' over jQuery's 'each.'
- Store jQuery objects in variables when used multiple times for improved efficiency.
const hoge = $(‘.JsHoge’);
if(hoge.hasClass(‘Test’)) {
hoge.removeClass(‘Test’);
}
- Use event delegation for multiple DOM elements
$(document).on(‘click’, ‘.JsHoge’ function() {
~
})
- Choose Intersection Observer for scroll-related tasks
- Use matchMedia for resizing-related tasks